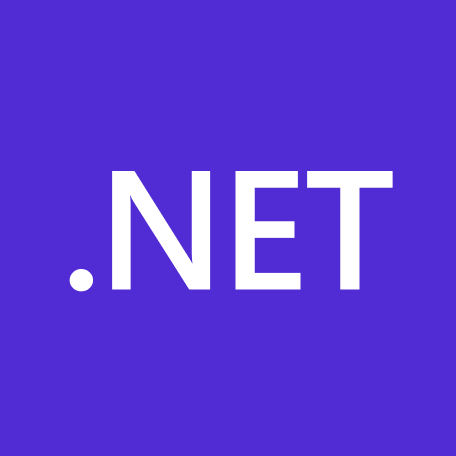
msdnのチュートリアルではSQL Serverを利用するようになっていますが、データベースを用意するのが面倒なので、データベースはSQLiteにします。
プロジェクトの作成
mkdir プロジェクト名
cd プロジェクト名
dotnet new mvc
dotnet add package Microsoft.AspNetCore.Diagnostics.EntityFrameworkCore -v 5.0.0
dotnet add package Microsoft.EntityFrameworkCore.Sqlite -v 5.0.0
dotnet add package Microsoft.EntityFrameworkCore.Tools -v 5.0.0
dotnet add package Microsoft.VisualStudio.Web.CodeGeneration.Design -v 5.0.0
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net5.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.Diagnostics.EntityFrameworkCore" Version="5.0.0" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Sqlite" Version="5.0.0" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Tools" Version="5.0.0">
<IncludeAssets>runtime; build; native; contentfiles; analyzers; buildtransitive</IncludeAssets>
<PrivateAssets>all</PrivateAssets>
</PackageReference>
<PackageReference Include="Microsoft.VisualStudio.Web.CodeGeneration.Design" Version="5.0.0" />
</ItemGroup>
</Project>
.Net8でも動作しました。nullがらみで警告が出ますがパッケージのバージョンを特別指定することなくサンプルプログラムの動作を確認しました。
Modelとコンテキストを作成
ModelsにPersonクラスを作成
using System;
using System.Collections.Generic;
namespace ContactList.Models
{
public class Person
{
public int ID { get; set; }
public string Name { get; set; }
}
}
多分データベースのテーブルと紐づくと思われます。
通常データベースのデータをプログラミング言語から操作する場合、データベースのテーブルの内容をプログラミング言語で扱いやすい変数と紐づける必要があります。紐づける作業が必要となるわけですが、これが結構定型的な作業になりがち(テーブルのNameフィールドをクラスのNameプロパティにセットするなど)でフィールド数が多くなると入力量が多くなります。といことでその作業をコンピュータに任せる仕組みにO/Rマッピングがあります。
EnitityFrameworkもこのO/Rマッピングが使われており、Entityはテーブルにアクセスするインターフェースオブジェクトとして機能します。またEntityをもとにテーブルを作成する際テーブルの設計図としても機能します。(Code Firstというらしい)
ということを調べて学びました。
Dataフォルダを作成しContactListContextクラスを作成
using ContactList.Models;
using Microsoft.EntityFrameworkCore;
namespace ContactList.Data
{
public class ContactListContext : DbContext
{
public ContactListContext(DbContextOptions<ContactListContext> options) : base(options)
{
}
public DbSet<Person> Persons { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Person>().ToTable("Person");
}
}
}
Startup.csにコンテキストの登録
using ContactList.Data; // 追加
using Microsoft.EntityFrameworkCore; // 追加
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.HttpsPolicy;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
namespace ContactList
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ContactListContext>(options =>
options.UseSqlite(Configuration.GetConnectionString("DefaultConnection"))); // 追加
services.AddDatabaseDeveloperPageExceptionFilter(); // 追加
services.AddControllersWithViews();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
}
}
またConfiguration.GetConnectionString(“DefaultConnection”)を引数にしていますので、こちらがデータベースの接続文字列だと思われます。
appsettings.jsonに接続文字列をセット
{
"ConnectionStrings": {
"DefaultConnection": "Data Source = C:/Users/karet/source/repos/ContactList/Sample.db"
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*"
}
後々を考えるとデータベースファイルは相対パスで記述したいところですが、どこにファイルが出来上がるか不明ですので絶対パスで記述しています。
DataフォルダにDbInitializerクラスを作成する
using ContactList.Models;
using System;
using System.Linq;
namespace ContactList.Data
{
public static class DbInitializer
{
public static void Initialize(ContactListContext context)
{
context.Database.EnsureCreated();
if (context.Persons.Any())
{
return; // DB has been seeded
}
var persons = new Person[]
{
new Person{ID=1, Name="Taro"},
new Person{ID=2, Name="Jiro"},
new Person{ID=3, Name="Hanako"},
};
foreach (var s in persons)
{
context.Persons.Add(s);
}
context.SaveChanges();
}
}
}
Program.csを変更する
using ContactList.Data; // 追加
using Microsoft.Extensions.DependencyInjection; // 追加
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Logging;
namespace ContactList
{
public class Program
{
public static void Main(string[] args) // 変更
{
var host = CreateHostBuilder(args).Build();
CreateDbIfNotExists(host);
host.Run();
}
private static void CreateDbIfNotExists(IHost host) // 追加
{
using (var scope = host.Services.CreateScope())
{
var services = scope.ServiceProvider;
try
{
var context = services.GetRequiredService<ContactListContext>();
DbInitializer.Initialize(context);
}
catch (Exception ex)
{
var logger = services.GetRequiredService<ILogger<Program>>();
logger.LogError(ex, "An error occurred creating the DB.");
}
}
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
}
}
実行してみる
ここまでmsdnのチュートリアルを進めてきましたが、これ以降はVisualStudioを使った作業となりますので、dotnet.exeとVSCodeを使っている関係でこのまま進めることが出来ません。とは言えここまでのプログラムでデータベースを作成はしてくれそうなので、とりあえずこの辺りでF5キーを押してデバッグ実行してみます。
実行したところEdgeが起動しMVCで出来たページが表示されました。データソースで指定したデータベースファイルが出来上がっていたので、思った通りに動いてくれました。
ビューとコントローラーを作成する
Controllers/PersonsController.csを作成
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
using Microsoft.EntityFrameworkCore;
using ContactList.Data;
using ContactList.Models;
namespace ContactList.Controllers
{
public class PersonsController : Controller
{
private readonly ContactListContext _context;
public PersonsController(ContactListContext context)
{
_context = context;
}
// GET: Persons
public async Task<IActionResult> Index()
{
return View(await _context.Persons.ToListAsync());
}
// GET: Persons/Details/5
public async Task<IActionResult> Details(int? id)
{
if (id == null)
{
return NotFound();
}
var person = await _context.Persons
.FirstOrDefaultAsync(m => m.ID == id);
if (person == null)
{
return NotFound();
}
return View(person);
}
// GET: Persons/Create
public IActionResult Create()
{
return View();
}
// POST: Persons/Create
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Create([Bind("ID,Name")] Person person)
{
if (ModelState.IsValid)
{
_context.Add(person);
await _context.SaveChangesAsync();
return RedirectToAction(nameof(Index));
}
return View(person);
}
// GET: Persons/Edit/5
public async Task<IActionResult> Edit(int? id)
{
if (id == null)
{
return NotFound();
}
var person = await _context.Persons.FindAsync(id);
if (person == null)
{
return NotFound();
}
return View(person);
}
// POST: Persons/Edit/5
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Edit(int id, [Bind("ID,Name")] Person person)
{
if (id != person.ID)
{
return NotFound();
}
if (ModelState.IsValid)
{
try
{
_context.Update(person);
await _context.SaveChangesAsync();
}
catch (DbUpdateConcurrencyException)
{
if (!PersonExists(person.ID))
{
return NotFound();
}
else
{
throw;
}
}
return RedirectToAction(nameof(Index));
}
return View(person);
}
// GET: Persons/Delete/5
public async Task<IActionResult> Delete(int? id)
{
if (id == null)
{
return NotFound();
}
var person = await _context.Persons
.FirstOrDefaultAsync(m => m.ID == id);
if (person == null)
{
return NotFound();
}
return View(person);
}
// POST: Persons/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public async Task<IActionResult> DeleteConfirmed(int id)
{
var person = await _context.Persons.FindAsync(id);
_context.Persons.Remove(person);
await _context.SaveChangesAsync();
return RedirectToAction(nameof(Index));
}
private bool PersonExists(int id)
{
return _context.Persons.Any(e => e.ID == id);
}
}
}
ControllerのpublicのメソッドがブラウザなどのWebクライアントからアクセスされるURLに対応します。
そのメソッド内でViewをレンダリングに必要な情報を用意しViewのレンダリングに処理を呼び出して終了するような感じです。
個人的に見た目以外のビジネスロジックを組み込みたくなりますが、MCVの考え方からするとModelとViewの仲介役に徹する方が良さそうです。Viewは見た目を司りますので、画面を構成する必要なデータはControllerから受け取りViewでは画面の作成に専念した方が良さそうです。
そうなりますと、ビジネスロジックはModelのお仕事になります。ModelをクラスライブラリなどのASPに依存しない形で作成するとテストプログラムが書きやすいですし、他のプロジェクト(デスクトップアプリケーションなど)に再利用することも出来ます。
データソースがDB以外だったり、DBでもWebAPIを介してデータへアクセスする場合なども考えられますので、Modelの中でもビジネスロジックとデータソースとのアクセス部分を分離した方が良さそうな感じがします。
Views/Shared/_Layout.cshtmlを変更
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>@ViewData["Title"] - ContactList</title>
<link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.min.css" />
<link rel="stylesheet" href="~/css/site.css" />
</head>
<body>
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-area="" asp-controller="Home" asp-action="Index">ContactList</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Persons" asp-action="Index">Persons</a> @* 変更 *@
</li>
</ul>
</div>
</div>
</nav>
</header>
<div class="container">
<main role="main" class="pb-3">
@RenderBody()
</main>
</div>
<footer class="border-top footer text-muted">
<div class="container">
© 2021 - ContactList - <a asp-area="" asp-controller="Home" asp-action="Privacy">Privacy</a>
</div>
</footer>
<script src="~/lib/jquery/dist/jquery.min.js"></script>
<script src="~/lib/bootstrap/dist/js/bootstrap.bundle.min.js"></script>
<script src="~/js/site.js" asp-append-version="true"></script>
@await RenderSectionAsync("Scripts", required: false)
</body>
</html>
PersonsコントローラーのIndexアクションが呼び出されるように変更します。
cshtmlはRazorマークアップエンジンでHTMLをレンダリングします。基本@がC#でそれ以外がHTMLといった感じです。
その中で_Layout.cshtmlはビューの共通部分でHTMLヘッダーやフッター及びBodyのメニューなど定義していますので、どのページでも表示してほしい内容を記述するとよさそうです。
@部分を眺めるとHTMLのtitleが@ViewData["Title"]となっていますので、その変数の値がHTMLのtitleとなると思われます。
@ViewData["Title"]
@RenderBody()は名称からここにHTMLのBODYをレンダリングすると思われます。
@RenderBody()
@await RenderSectionAsync("Scripts", required: false)は調べて所、Scriptsという名前のセクションを非同期にレンダリングするようです。セクションが何を指示しているか今のところ不明です。
@await RenderSectionAsync("Scripts", required: false)
またHTML構文の中に見れない文字列があります。
<a class="navbar-brand" asp-area="" asp-controller="Home" asp-action="Index">ContactList</a>
asp-から始まる文字列はアンカータグヘルパーというものみたいです。HTMLでは以下のようになりました。
<a class="navbar-brand" href="/">ContactList</a>
hrefの中身に対応するようですが、/Home/Indexが省略されて/に変換されているようです。asp-areaは何をしているか今の段階で不明です。
変更した部分をリンクを見てみます。
<a class="nav-link text-dark" asp-area="" asp-controller="Persons" asp-action="Index">Persons</a> @* 変更 *@
<a class="nav-link text-dark" href="/Persons">Persons</a>
ActionのIndexが省略されていますが、asp-controllerとasp-actionが/Persons(コントローラー)/Index(アクション)に変換されているようです。
_Layout.cshtmlは各ビューと組み合わされて完成するため、それ単体で見ると不明な点があります。
Views/Persons/Index.cshtmlを作成
@model IEnumerable<ContactList.Models.Person>
@{
ViewData["Title"] = "Index";
}
<h1>Index</h1>
<p>
<a asp-action="Create">Create New</a>
</p>
<table class="table">
<thead>
<tr>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th></th>
</tr>
</thead>
<tbody>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
<a asp-action="Edit" asp-route-id="@item.ID">Edit</a> |
<a asp-action="Details" asp-route-id="@item.ID">Details</a> |
<a asp-action="Delete" asp-route-id="@item.ID">Delete</a>
</td>
</tr>
}
</tbody>
</table>
@model IEnumerable<ContactList.Models.Person>
IEnumerableインターフェイスでContactList.Models.Personを要素をもつforeach可能なコレクションで変数名前がmodelのように見えます。
// GET: Persons
public async Task<IActionResult> Index()
{
return View(await _context.Persons.ToListAsync());
}
Viewを呼び出しているコントローラーのアクション部分を見ると、PoersonsをToListAsync()でListに変換してViewに渡しています。
後半でforeachが出てきます。
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
<a asp-action="Edit" asp-route-id="@item.ID">Edit</a> |
<a asp-action="Details" asp-route-id="@item.ID">Details</a> |
<a asp-action="Delete" asp-route-id="@item.ID">Delete</a>
</td>
</tr>
}
こちらがHTMLではこのようになります。
<table class="table">
<thead>
<tr>
<th>
Name
</th>
<th></th>
</tr>
</thead>
<tbody>
<tr>
<td>
Jiro
</td>
<td>
<a href="/Persons/Edit/2">Edit</a> |
<a href="/Persons/Details/2">Details</a> |
<a href="/Persons/Delete/2">Delete</a>
</td>
</tr>
<tr>
<td>
Hanako
</td>
<td>
<a href="/Persons/Edit/3">Edit</a> |
<a href="/Persons/Details/3">Details</a> |
<a href="/Persons/Delete/3">Delete</a>
</td>
</tr>
<tr>
<td>
Taro1
</td>
<td>
<a href="/Persons/Edit/4">Edit</a> |
<a href="/Persons/Details/4">Details</a> |
<a href="/Persons/Delete/4">Delete</a>
</td>
</tr>
</tbody>
</table>
foreachで3レコード分のテーブル作成されています。
asp-route-idは/コントロール/アクション/IDのIDに対応しURLの一部にIDを含めることでコントロールのアクションの引数として使うことが出来ます。
前後しますが@Html.DisplayNameFor()はPerson.Nameの名称のNameが@Html.DisplayFor()ではPerson.Nameの値が表示されています。
Views/Persons/Create.cshtmlを作成
@model ContactList.Models.Person
@{
ViewData["Title"] = "Create";
}
<h1>Create</h1>
<h4>Student</h4>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Create">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<input asp-for="Name" class="form-control" />
<span asp-validation-for="Name" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Create" class="btn btn-primary" />
</div>
</form>
</div>
</div>
<div>
<a asp-action="Index">Back to List</a>
</div>
@section Scripts {
@{await Html.RenderPartialAsync("_ValidationScriptsPartial");}
}
HTMLのform部分が出てきました。
<div class="row">
<div class="col-md-4">
<form asp-action="Create">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<input asp-for="Name" class="form-control" />
<span asp-validation-for="Name" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Create" class="btn btn-primary" />
</div>
</form>
</div>
</div>
実際のHTMLでは以下のように変換されていました。
<div class="row">
<div class="col-md-4">
<form action="/Persons/Create" method="post">
<div class="form-group">
<label class="control-label" for="Name">Name</label>
<input class="form-control" type="text" data-val="true" data-val-required="The Name field is required." id="Name" name="Name" value="" />
<span class="text-danger field-validation-valid" data-valmsg-for="Name" data-valmsg-replace="true"></span>
</div>
<div class="form-group">
<input type="submit" value="Create" class="btn btn-primary" />
</div>
<input name="__RequestVerificationToken" type="hidden" value="CfDJ8AV2kdxPuytLuv1jKhKI60r9K7nwexvUwrb-MMIgWO6GMJmvYF3w0CCPfFOtSLCQ2W5PZmZgycpTuMHbqu4Yg5JUFQRtgPZIIkGRjhC7M9bNszMFHNio3R8zrYTuzeAhqw_2XGc-dkv8j_NyFCacmlg" /></form>
</div>
</div>
cshtmlには見当たらないformのmethod=”post”が出現しました。あとinputの__RequestVerificationTokenが出現しました。
値がランダムな文字列のように見えるので、セッションなどプログラムを組む上で特別意識する必要のない部分かもしれません。
formで引数に見えるのはNameと謎の__RequestVerificationTokenぐらいで、それを受けるpost先のコントローラーのアクションではperson型のオブジェクトを引数として受け取っており、このあたりも謎です。
ちょっと見慣れないものがいろいろあって理解しがたいですが、バリデーションも行うように記述されているようにも見えます。
ただ、sqliteのデータベースに追加されたIDは別の数値がセットされており、特別テーブルのキー項目にセットした記憶がありませんが、IDがキー設定されておりかつ自動採番しているようです。
腑に落ちないので調べてみたところ、
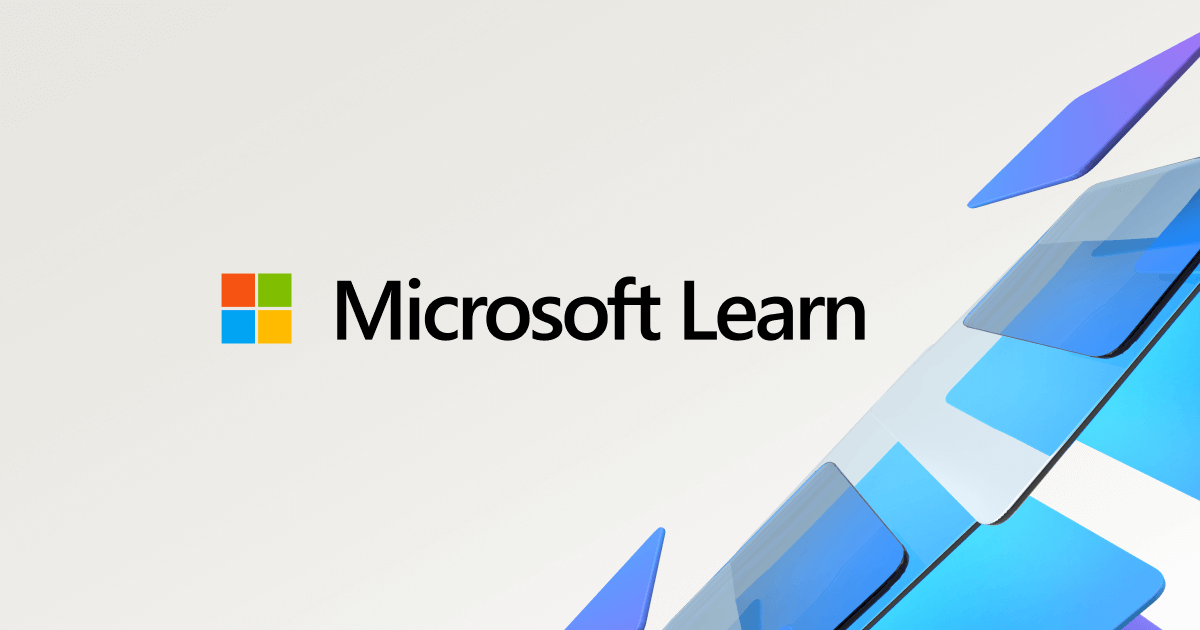
主キーの構成
慣例により、Id または >type name<Id という名前のプロパティがエンティティの主キーとして構成されます。
とのことです。
自動的にキーを設定し採番してくれるのは便利かもしれませんが、なんともしっくりこない感じがします。また、キーの無いテーブルも作る方法が別にあるようです。
@section Scripts {
@{await Html.RenderPartialAsync("_ValidationScriptsPartial");}
}
こちらは_Layout.cshtmlのRenderSectionAsync()に対応しSectionの名前がScritpであると推測すます。
_ValidationScriptsPartial.cshtmlが存在しますので、内容を非同期にレンダリングしてると思われますが、非同期であることがどういうことなのかは理解できていません。
Views/Persons/Delete.cshtmlを作成
@model ContactList.Models.Person
@{
ViewData["Title"] = "Delete";
}
<h1>Delete</h1>
<h3>Are you sure you want to delete this?</h3>
<div>
<h4>Person</h4>
<hr />
<dl class="row">
<dt class = "col-sm-2">
@Html.DisplayNameFor(model => model.Name)
</dt>
<dd class = "col-sm-10">
@Html.DisplayFor(model => model.Name)
</dd>
</dl>
<form asp-action="Delete">
<input type="hidden" asp-for="ID" />
<input type="submit" value="Delete" class="btn btn-danger" /> |
<a asp-action="Index">Back to List</a>
</form>
</div>
Views/Persons/Details.cshtmlを作成
@model ContactList.Models.Person;
@{
ViewData["Title"] = "Details";
}
<h1>Details</h1>
<div>
<h4>Person</h4>
<hr />
<dl class="row">
<dt class = "col-sm-2">
@Html.DisplayNameFor(model => model.Name)
</dt>
<dd class = "col-sm-10">
@Html.DisplayFor(model => model.Name)
</dd>
</dl>
</div>
<div>
<a asp-action="Edit" asp-route-id="@Model.ID">Edit</a> |
<a asp-action="Index">Back to List</a>
</div>
Views/Persons/Edit.cshtmlを作成
@model ContactList.Models.Person
@{
ViewData["Title"] = "Edit";
}
<h1>Edit</h1>
<h4>Person</h4>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Edit">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<input type="hidden" asp-for="ID" />
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<input asp-for="Name" class="form-control" />
<span asp-validation-for="Name" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Save" class="btn btn-primary" />
</div>
</form>
</div>
</div>
<div>
<a asp-action="Index">Back to List</a>
</div>
@section Scripts {
@{await Html.RenderPartialAsync("_ValidationScriptsPartial");}
}

エンティティ(テーブル)は大分省略しましたが、VSCodeとdotnet.exeの組み合わせで動くサンプルが出来上がりました。
初めにも書きましたがチュートリアルではデータベースがSQL Server(localdb)でしたが、こちらのサンプルではSQLiteにしてみました。
O/RマッピングはSQLを実行し結果をC#のオブジェクトへ変換(又はその逆)を自動化及びSQL部分を隠蔽することが出来ます。DBのテーブルで多量に存在する項目を一つ一つオブジェクトのプロパティなどにセットする処理はコードを書いていて楽しい処理ではなく、普段使わないリフレクションなどの機能を使ってでも自動化させたい気持ちにさせられます。
C#のコーディング的にはO/Rマッパーがあると大変楽が出来るのですが、DBのアクセスを共通化するためにテーブル全体を取得するプログラムを見たことがあります。
本来不要な項目もDBから取得しC#で絞り込むような形になってしまい、パフォーマンスが悪くなっているシステムを見たことがあります。
また、複数のテーブルをキー項目などでJoinするクエリなどもO/Rマッピングでは表現が難しいと思われます。SQLを使いたい場合DB側のView機能などにSQLを隠蔽すると良さそうな感じがします。
コメント