ソースコード
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="SubPageSample.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30,0"
VerticalOptions="Center">
<Label
Text="メインページ"
FontSize="32"
HorizontalOptions="Center" />
<Label
x:Name="Id"
Text=""
FontSize="32"
HorizontalOptions="Center" />
<Button
x:Name="SubBtn"
Text="サブページへ"
Clicked="OnSubBtnClicked"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
ファイル名:MainPage.xaml.cs
namespace SubPageSample;
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
this.Loaded += (s, e) => {
var card = new Card { Id = 0, };
(App.Current as App).Card = card;
Id.Text = String.Format("Card.Id:{0}", card.Id);
};
}
private async void OnSubBtnClicked(object sender, EventArgs e)
{
var page = new SubPage();
await Navigation.PushModalAsync(page);
var card = (App.Current as App).Card;
Id.Text = String.Format("Card.Id:{0}", card.Id);
}
}
ファイル名:SubPage.xaml
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="SubPageSample.SubPage"
Title="SubPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30,0"
VerticalOptions="Center">
<Label
Text="サブページ"
FontSize="32"
HorizontalOptions="Center" />
<Label
x:Name="Id"
Text=""
FontSize="32"
HorizontalOptions="Center" />
<Button
x:Name="MainBtn"
Text="メインページへ"
Clicked="OnMainBtnClicked"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
ファイル名:SubPage.xaml.cs
namespace SubPageSample;
public partial class SubPage : ContentPage
{
public SubPage()
{
InitializeComponent();
var card = (App.Current as App).Card;
this.Loaded += (s, e) =>
{
card.Id++;
this.Id.Text = String.Format("Card.Id:{0}", card.Id);
};
}
private async void OnMainBtnClicked(object sender, EventArgs e)
{
await Navigation.PopModalAsync();
}
}
ファイル名:App.xaml.cs
namespace SubPageSample;
public partial class App : Application
{
public App()
{
InitializeComponent();
MainPage = new AppShell();
}
public Card Card { get; set; }
}
ファイル名:Card.cs
namespace SubPageSample;
public class Card
{
public int Id { get; set; } = -1;
}
実行
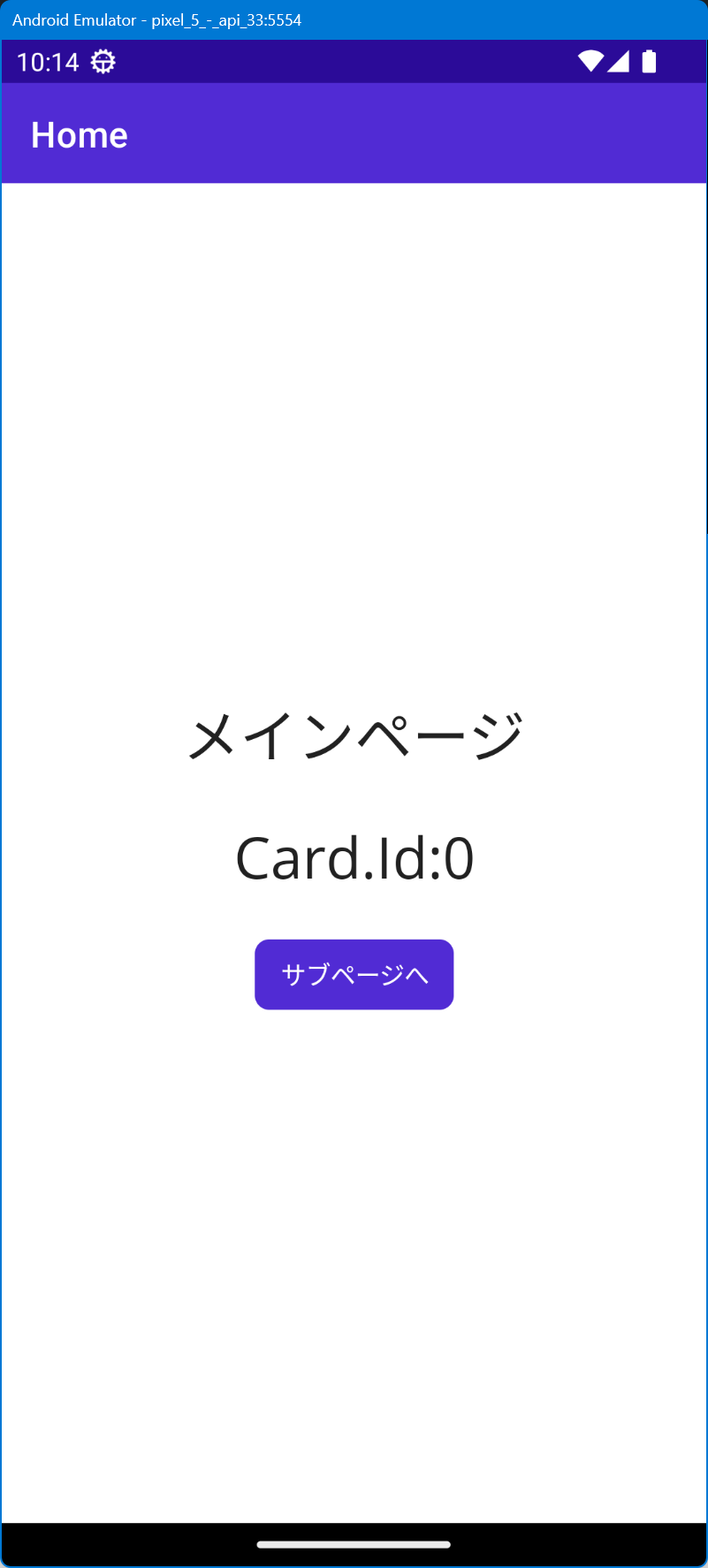
「サブページへ」ボタンをクリック
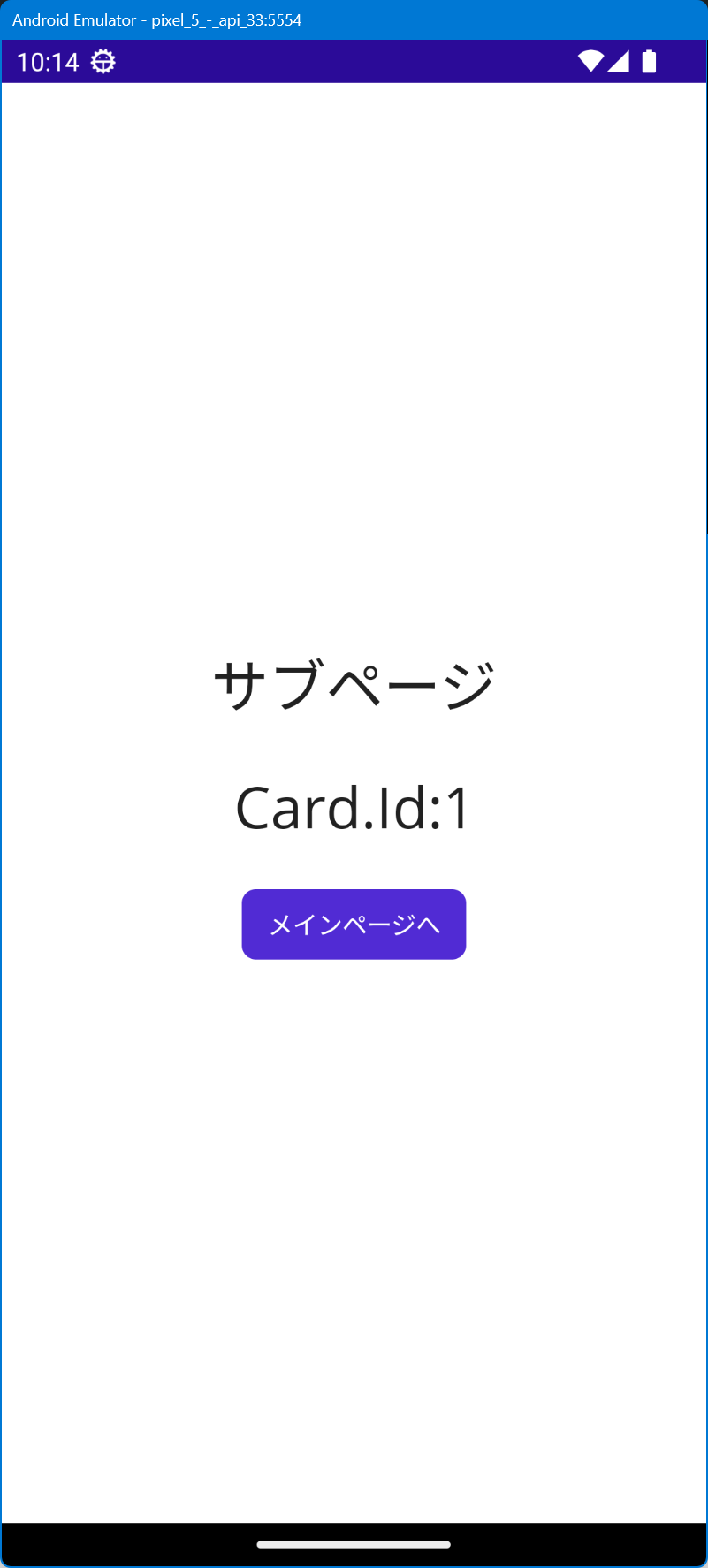
サブページが表示される。Card.Idをカウントアップ。「メインページへ」ボタンをクリック。
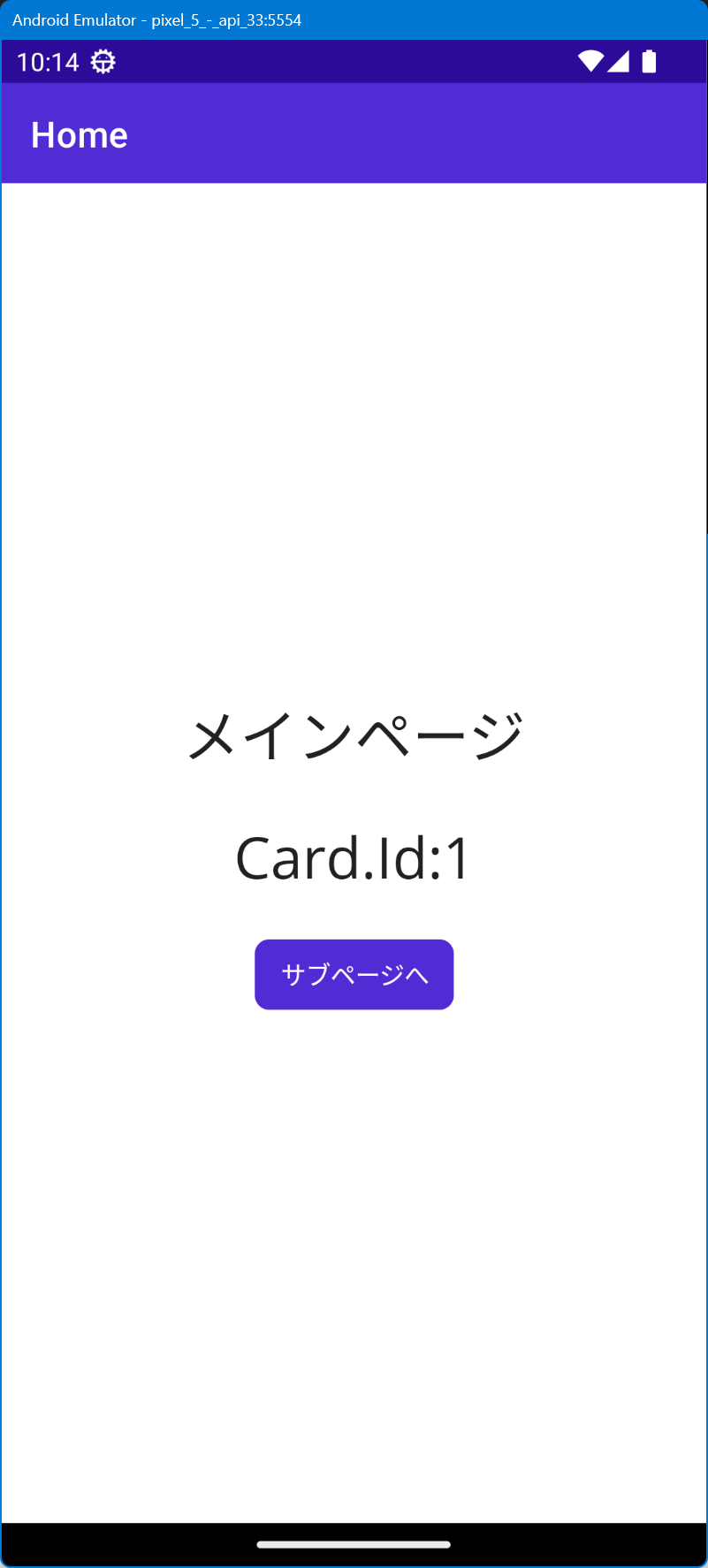
メインページへ戻ってきます。サブページでカウントアップしたIdが反映されています。
説明
呼び出し先のサブページから呼び出し元のメインページに戻る場合Navigation.PopModalAsync();を呼び出します。
ページ間でデータを共有するために、App()にCardクラスのオブジェクトをプロパティとして定義しています。各ページからは(App.Current as App).Cardの形式でプロパティにアクセスしています。
今回はCardという普通のクラスを作成してみましたが、実用的にはViewModelを共有するとよさそうです。
コメント