プロジェクトの作成
PowerShellで実行。要dotnet.exe
mkdir Clock3
cd Clock3
dotnet new winforms
code .
ソースコード
ファイル名:Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Clock3
{
public partial class Form1 : Form
{
PictureBox picbox1 = new PictureBox
{
Dock = DockStyle.Fill,
};
Button button1 = new Button
{
Dock = DockStyle.Bottom,
Text = "Start",
Height = 80,
};
Bitmap bmp = null;
Font fnt = new Font("MS UI Gothic", 48);
System.Threading.Timer timer;
void timerCallBack(Object state)
{
if (picbox1 == null) return;
if (picbox1.InvokeRequired)
{
Invoke(new Action(() =>
{
lock(this)
{
if (this.bmp == null)
{
this.bmp = new Bitmap(this.picbox1.Width, this.picbox1.Height);
}
using(var g = Graphics.FromImage(this.bmp))
{
DateTime dt = DateTime.Now;
string text = dt.ToString("HH:mm:ss");
SizeF stringSize = g.MeasureString(text, fnt);
int x = (bmp.Width - stringSize.ToSize().Width) / 2;
int y = (bmp.Height - stringSize.ToSize().Height) / 2;
g.FillRectangle(
Brushes.White, x, y, stringSize.ToSize().Width, stringSize.ToSize().Height);
g.DrawString(text, fnt, Brushes.Black, x, y);
}
this.picbox1.Invalidate();
}
}));
}
}
public Form1()
{
InitializeComponent();
this.Controls.Add(button1);
this.Controls.Add(picbox1);
this.timer = new System.Threading.Timer(
timerCallBack, null, Timeout.Infinite, Timeout.Infinite);
this.picbox1.Resize += (s, e) =>
{
lock(this)
{
if (this.bmp != null)
{
this.bmp.Dispose();
this.bmp = null;
}
}
};
this.picbox1.Paint += (s, e) =>
{
lock(this)
{
if (this.bmp != null)
{
e.Graphics.DrawImageUnscaled(this.bmp, 0, 0);
}
}
};
this.FormClosing += (s, e) =>
{
if (this.timer != null)
{
this.timer.Change(Timeout.Infinite, Timeout.Infinite);
this.timer.Dispose();
}
};
this.button1.Click += (s, e) =>
{
if (this.button1.Text == "Start")
{
this.button1.Text = "Stop";
this.timer.Change(0, 1000);
}
else
{
this.button1.Text = "Start";
this.timer.Change(Timeout.Infinite, Timeout.Infinite);
}
};
}
}
}
実行
「Start」ボタンを押すと時計が表示されます。
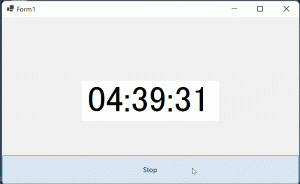
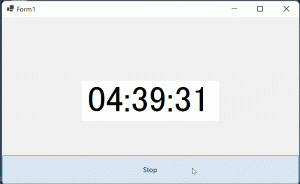
System.Threading.Timer
を使い1秒(1000ミリ秒)ごとにPictureBox
の画像に時刻の文字列を描画しています。
コメント