namespace AsyncBitmap;
public partial class Form1 : Form
{
FlowLayoutPanel _basePanel = new()
{
Dock = DockStyle.Fill,
};
PictureBox _picbox = new()
{
Size = new Size(512, 384),
SizeMode = PictureBoxSizeMode.Zoom,
};
Button _syncButton = new()
{
Text = "同期",
AutoSize = true,
};
Button _asyncButton = new()
{
Text = "非同期",
AutoSize = true,
};
public Form1()
{
InitializeComponent();
this.Load += Form1_Load;
}
void Form1_Load(Object? o, EventArgs e)
{
_basePanel.Controls.AddRange(new Control[]
{
_picbox,
_syncButton,
_asyncButton,
});
this.Controls.Add(_basePanel);
_basePanel.SetFlowBreak(_picbox, true);
var path = @"読み込む画像ファイルのパス";
_syncButton.Click += (s, e) =>
{
Task.Delay(1000).Wait();
using var fs = new FileStream(path, FileMode.Open, FileAccess.Read);
Image img = Bitmap.FromStream(fs);
_picbox.Image?.Dispose();
_picbox.Image = img;
};
_asyncButton.Click += async (s, e) =>
{
await Task.Delay(1000);
using var fs = new FileStream(path, FileMode.Open, FileAccess.Read);
Image img = await Task.Run(()=>Bitmap.FromStream(fs));
_picbox.Image?.Dispose();
_picbox.Image = img;
};
}
}
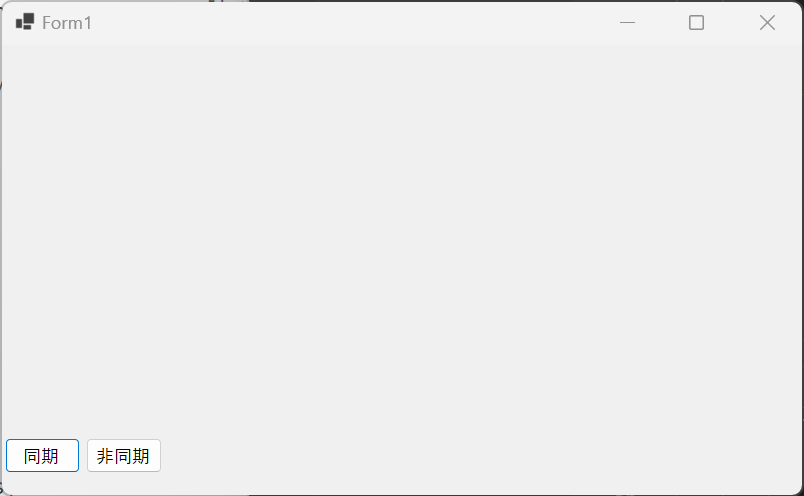
「同期」ボタンを押すと画像が表示されるまで、ボタンなどのUIがロックされます。(Task.Delay()でウエイトを入れています。)
「非同期」ボタンの場合はロックされません。
一応思った通りになりましたが、これでよいのか…もっと調べてみたいと思います。
コメント