ソースコード
<Window x:Class="ListBoxSample1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:ListBoxSample1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<StackPanel Margin="10" >
<ListBox x:Name="listbox1" />
<WrapPanel
Orientation="Horizontal"
Margin="10">
<Button x:Name="addButton" Content="追加" />
<Button x:Name="delButton" Content="削除" />
<Button x:Name="upButton" Content="上へ" />
<Button x:Name="downButton" Content="下へ" />
</WrapPanel>
</StackPanel>
</Grid>
</Window>
ファイル名:MainWindow.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Collections.ObjectModel;
using System.Diagnostics;
namespace ListBoxSample1
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
ObservableCollection<string> _list = new();
public MainWindow()
{
InitializeComponent();
listbox1.ItemsSource = _list;
// ロード後
Loaded += (s, e) =>
{
_list.Add("1行目");
_list.Add("2行目");
_list.Add("3行目");
};
// 追加
addButton.Click += (s, e) =>
{
_list.Add($"{_list.Count+1}行目");
};
// 削除
delButton.Click += (s, e) =>
{
// 選択中のインデックスを取得
var index = listbox1.SelectedIndex;
if (index == -1) return; // 未選択
// コレクションからインデクス指定で要素を削除
_list.RemoveAt(index);
Debug.Print($"index:{index}");
};
// 上へ移動
upButton.Click += (s, e) =>
{
var index = listbox1.SelectedIndex;
if (index < 1) return; // 移動不可
// 要素の移動
_list.Move(index, index-1);
};
// 下へ移動
downButton.Click += (s, e) =>
{
var index = listbox1.SelectedIndex;
if (index < 0) return; // 未選択
if (index >= (_list.Count-1)) return; // 移動不可
// 要素の移動
_list.Move(index, index+1);
};
// 選択要素が変更された
listbox1.SelectionChanged += (s, e) =>
{
var index = listbox1.SelectedIndex;
if (index < 0) return;
this.Title = $"{_list[index]}が選択された。";
};
}
}
}
実行
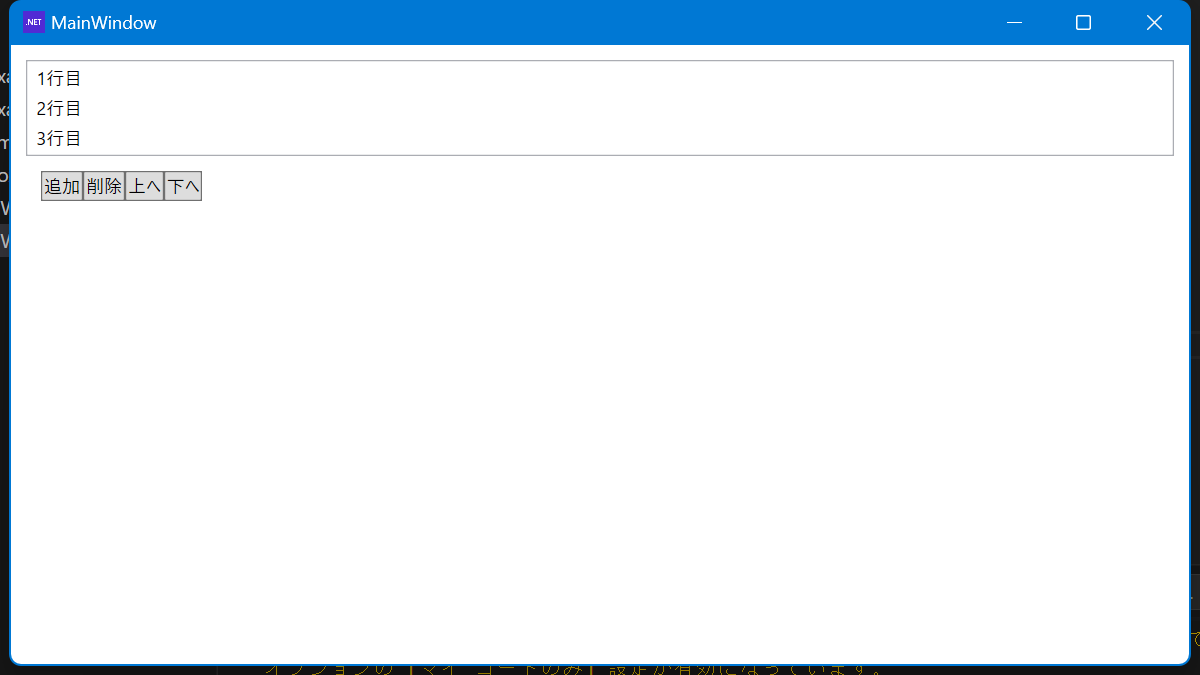
listboxの要素を選択するとタイトルに選択した要素が表示されます。
ObservableCollectionの要素をstring型
にしていますが、通常クラスを定義してそのクラスを指定します。
その場合XAML側でListBoxのプロパティDisplayMemberPathで表示項目、SelectedValuePathで選択値の項目を定義したクラスのプロパティから指定します。
C#でWPF学習中「ReactiveCollectionとListbox」WPFでReactiveCollectionのオブジェクトをListboxのデータソースとしてバインドする方法を試してみました。ReactiveCollectionを使うことでUI側の制約をあまり気にすることなく要素の追加削除移動が出来るこ...
コメント