WPFでBitmap画像に対しピクセル単位で描画する方法を探したところ、byte配列でピクセルデータを用意し、そのbyte配列をWriteableBitmapオブジェクトに書き込む方法が見つかりましたので試してみます。
プロジェクトの作成
PowerShellで実行。要dotnet.exe
mkdir WriteableBitmapSample
cd WriteableBitmapSample
dotnet new wpf
code .
ソースコード
<Window x:Class="WriteableBitmapSample.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WriteableBitmapSample"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<Image x:Name="image1" Stretch="None" />
</Grid>
</Window>
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace WriteableBitmapSample
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
const int width = 256;
const int height = 256;
var wb = new WriteableBitmap(width, height, 75, 75, PixelFormats.Bgra32, null);
int pixelsSize = (width * 4) * height;
byte[] pixels = new byte[pixelsSize];
for (int y = 0; y < height; y++)
{
for(int x = 0; x < width; x++)
{
int v = x + (width * y);
int i = (x * 4) + (y * (width * 4));
//byte b = (byte)((v & 0xFF0000) / (256 * 256));
byte b = 0xFF;
byte g = (byte)((v & 0x00FF00) / 256);
byte r = (byte)(v & 0x0000FF);
byte a = 0xFF;
pixels[i] = b;
pixels[i+1] = g;
pixels[i+2] = r;
pixels[i+3] = a;
}
}
wb.WritePixels(new Int32Rect(0, 0, width, height), pixels, (width * 4), 0, 0);
image1.Source = wb;
}
}
}
ビルド
dotnet build
実行
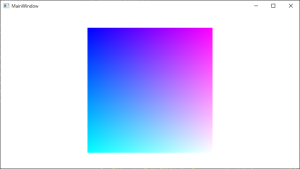
なるべく沢山の色が表示されるようにしてみました。
コメント